Calculator app has been around in macOS forever. Here's how to use its four modes in macOS Sequoia.
Apple's Calculator app lives in the /Applications folder at the root of your Startup Disk. One of the simplest of all the macOS apps, it was also one of the first utilities shipped with Mac OS X when it was released in 2000.
In fact, the original Mac also shipped with a very simple Calculator app as a desk accessory.
The original calculator was extremely simple: with just one tiny window. Other than some minor cosmetic user interface changes, Calculator has remained largely unchanged over the years.
Four Calculator modes
Mac OS X introduced additional modes to expand Calculator's features. These modes are:
- Basic
- Scientific
- Programmer
- Convert
To change modes in macOS Sequoia's Calculator, launch the app in the Finder, then click the small calculator icon in the lower left corner:
In Basic mode, you get what the Calculator has always been: a simple single window for performing basic calculations.
Scientific mode provides a wider interface with more buttons and a lot of standard scientific formula buttons, just as you would find on a real physical scientific calculator.
This mode provides all the buttons found in Basic mode, but it also adds memory, squaring, random, logarithmic, sine/cosine/tangent, Pi, and more.
Programmer mode
In Programmer mode, you also get a wider UI, but now you also get a host of buttons useful for common programming calculations including:
- Boolean logical operators
- Bitwise operators
- Bit-shifting
- 2's compliment (negation)
- Modulo (remainder)
- Rotate
- Byte flipping
- Base (octal, decimal, or hexadecimal) display
- Binary display toggle
- ASCII or Unicode
- Hex input (FF and A-F)
- Parenthesis (precedence)
- Reverse Polish Notation (RPN)
Base 10 (decimal) is the numeric representation you're familiar with: numbers 1-10.
Hexadecimal (Base 16) is a numbering system that uses decimal numbering for numbers 1-10 but extends the decimal numbering system to 16 by using the letters A-F (the first six letters of the alphabet). In hexadecimal you'll see numbers and A-F combined to represent one base-16 number.
In many programming languages, hexadecimal (sometimes simply called 'hex') numbers are prefixed with an '0x' to indicate they're being displayed as hex.
Logical and bitwise operations
Logical, or Boolean operators are used for evaluating the truth of statements. Logical operators return either 0 or 1, depending on whether the expression being evaluated is true or false. These include:
- AND
- OR
- XOR (eXclusive Or)
- NOT
- NOR (Negation of Or)
In most languages, logical operations can be nested into compound statements, although doing so can become confusing very quickly if the statement is complex.
Bitwise operators can take one (unary), two (binary), or three (tertiary) elements, perform evaluations on them, and produce a result. Bitwise operations can also be nested to perform compound evaluations.
For example in the C programming language to increment the value of variable 'x' using the unary operator you'd simply write:
x++;
This is the same as using:
x = ( x + 1 );
To decrement the same variable you'd use the '— ' operator:
x--;
There are other kinds of bitwise operators in most languages including the simple math operators you're already familiar with: +, -, * (multiplication), / (division), % (remainder).
Relational bitwise operators evaluate two or more numbers or statements for value. Again, using C as an example: , =, == (equal), != (not equal).
Boolean logic
Numbers or expressions can also be logically evaluated at the numeric level using numeric logical operators: && (AND), || (OR), ! (NOT) in C. These can be expressed in the macOS Calculator by using the logical Boolean buttons mentioned above.
A code example using the numerical OR operator in C might be:
if ( ( x || y ) == 1 )
{
// Do something
}
This is an example of a compound statement evaluation in which the values of variables x and y are checked against the value of '1' and if either is true, the "Do something" code section would run.
The same evaluation could be made using the AND (&&) operator to check the truth of both x and y:
if ( ( x && y ) == 1 )
Actually, this code may be a little misleading, because in this case, the statement will evaluate to true if both x and y contain any non-zero numbers. The same is true of the OR case above - if either x or y is non-zero the result will be true (1).
To specifically check both x and y for the value '1', you'd have to use two more sets of parenthesis:
if ( ( x == 1 ) && ( y == 1 )
{
// Do something
}
The '=='
relational operator means "is equal to" in C.
For Boolean (true/false) comparisons, C doesn't include a boolean data type, but most compilers have since added a typedef
enum using the names 'true' and 'false' (or 'TRUE' and 'FALSE') which are assigned zero, and non-zero, respectively - but as a C bool
type rather than numbers:
typedef enum {false, true} bool;
ANSI C99 added the Boolean
type in the stdbool.h
header file. So you could, instead write the above if
statement as:
if ( ( x == true ) && ( y == true )
Actually, C defines any non-zero value as 'true'. Only zero means 'false'.
In C and in many other languages parenthesis are used to indicate the order or precedence of evaluation. You can use the parenthesis keys in the macOS Calculator to build compound statements for evaluation just as you would in code.
Statements enclosed in parenthesis are evaluated from the most deeply nested statements outwards.
Finally, single bitwise operators can be used to evaluate the bits in numbers or in results of statements themselves: &, |, >, ~, ^.
For example, the '&' operator takes two numbers and does a logical AND on every bit in both numbers. The result is true only if both bits are the same.
Don't worry if you don't understand hex numbers, Boolean logic, and bitwise operators right away - it takes some getting used to. Eventually, with practice, you'll get it.
Byte flipping
Modern CPUs order their 8-bit and 16-bit values in memory in different patterns. Some CPUs (such as x86) use Little Endian ordering, while others (such as PowerPC) use Big Endian.
Byte flipping can be used to flip half of each 8 or 16-bit number to the opposite "Endian-ness". The Calculator app has two buttons for performing such byte-flipping, or byte-swapping: Flip8 and Flip16.
Clicking either button on any 8-bit or 16-bit number will reverse the lower and upper halves of the number. It's easier to visualize this in hexadecimal format. For example, flipping the 8-bit (one-byte) value 0xABCD
becomes:
0xCDAB
Flipping a 16-bit (two-byte) number reverses the upper and lower bytes without flipping each 8-bit half. For example 0xABCD1234
becomes:
0x1234ABCD
To flip all halves of a 16-bit value and its two 8-byte halves you'd first have to flip both 8-bit halves, then flip the 16-bit result.
Byte-flipping may be necessary for example if you read values from a file written on one computer on another computer whose CPU has the opposite Endian-ness (for example reading a file written on an x86 machine on a PowerPC-based Mac). If you didn't flip the bytes after reading the file, you'd get garbled data that appears to be corrupted.
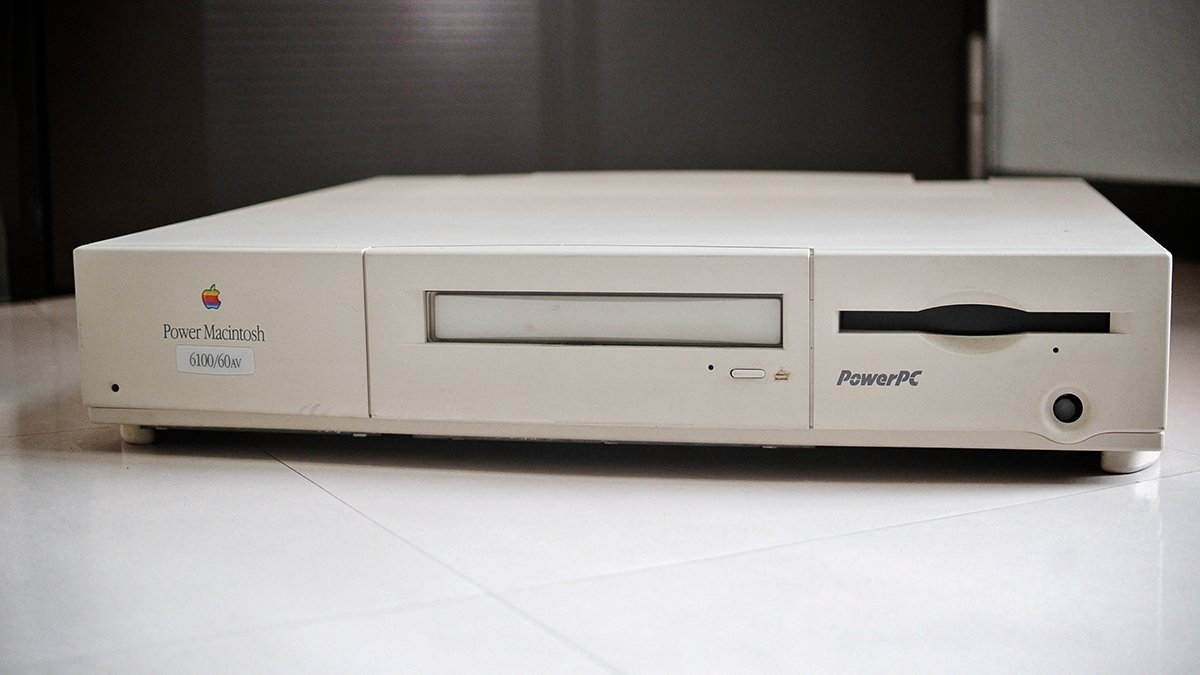
ASCII, Unicode and display buttons
At the top of the Calculator window in Programmer mode are two toggle buttons for displaying info in either ASCII (8-bit) or Unicode (16-bit) format, toggling the binary number display on or off, and the three buttons for setting the numerical format (8, 10, or 16). You use these buttons to change how numbers in Calculator are displayed.
Using conversions
In either Basic or Scientific mode, if the value in the main data entry field is non-zero, you can click the calculator icon button in the lower left corner again and select Convert in the popup menu.
This adds an additional section - and two small popup menus to the data entry field for conversions:
Conversion mode assumes currency conversion by default, but if you click either of the two additional popup menus in the data entry field, you can choose from angle, area, data, energy, and more.
You can also search for available unit types by using the search field.
Conversion mode allows you to easily switch between two calculations using different units of measurement:
There's also a history feature in Calculator that allows you to view past calculation operations by selecting View-> Show History from the menu bar.
The advanced features in macOS's Calculator app in Sequoia are easy to use and will help out a lot if you're a programmer or scientist. The new Calculator UI is simple to understand and is easier on the eyes.
Apple does have a Calculator User Guide on the Apple website, but it's currently a little limited and needs to be expanded a bit.
There are no Comments Here, Yet
Be "First!" to Reply on Our Forums ->